7DRL Day 2: Equipment, Combat (almost)
Yesterday I designed my first RPG combat system. It was hell. I'm really worried that I won't be able to make a balanced game out of it by Saturday even though it's pretty simple, but I'm sticking with it, because at this point I have no choice.
Each combatant has Strength and Reflex stats. They also have a ranged or melee weapon with a strength requirement, and armor covering head, body, and hands. (No legs or feet yet for simplicity). Ranged weapons have a "falloff" factor, which is the percentage hit chance they lose for every cell of distance to the target.
Here's some pseudocode that determines combat outcomes (link with nicer formatting):
let strengthFactor = ( attacker.stats.strength - attacker.weapon.strengthRequirement) if attacker.weapon.isMelee: hitChance = (1 - defender.stats.reflex) * strengthFactor else: hitChance = ( (1 - defender.stats.reflex) * (1 - (attacker.weapon.rangeFalloff * distance)) // too close for a ranged weapon to be accurate! if distance <= 1: hitChance /= 2 if random.random() > hitChance { return Outcome.miss() } let armor = defender.armor[random.choice(ARMOR_TYPES)] // there are 3 damage types: // physical, heat/fire, and electric. // each piece of armor has resistance values for each, // which determines damage. var total = 0 if weapon.isMelee: // physical weapon damage is affected by strength total += ( attacker.weapon.damagePhysical * strengthFactor * (1 - armor.physicalResistance)) else: total += attacker.weapon.damagePhysical * (1 - armor.physicalResistance) total += attacker.weapon.damageElectric * (1 - armor.electricResistance) total += attacker.weapon.damageHeat * (1 - armor.heatResistance) // Result: A number roughly between 0 and 1 that I can multiply by an arbitrary scaling factor
I've written the code to calculate how each round of combat will go, and it runs whenever you mouse over an enemy. Check out the lower right side of this screenshot:
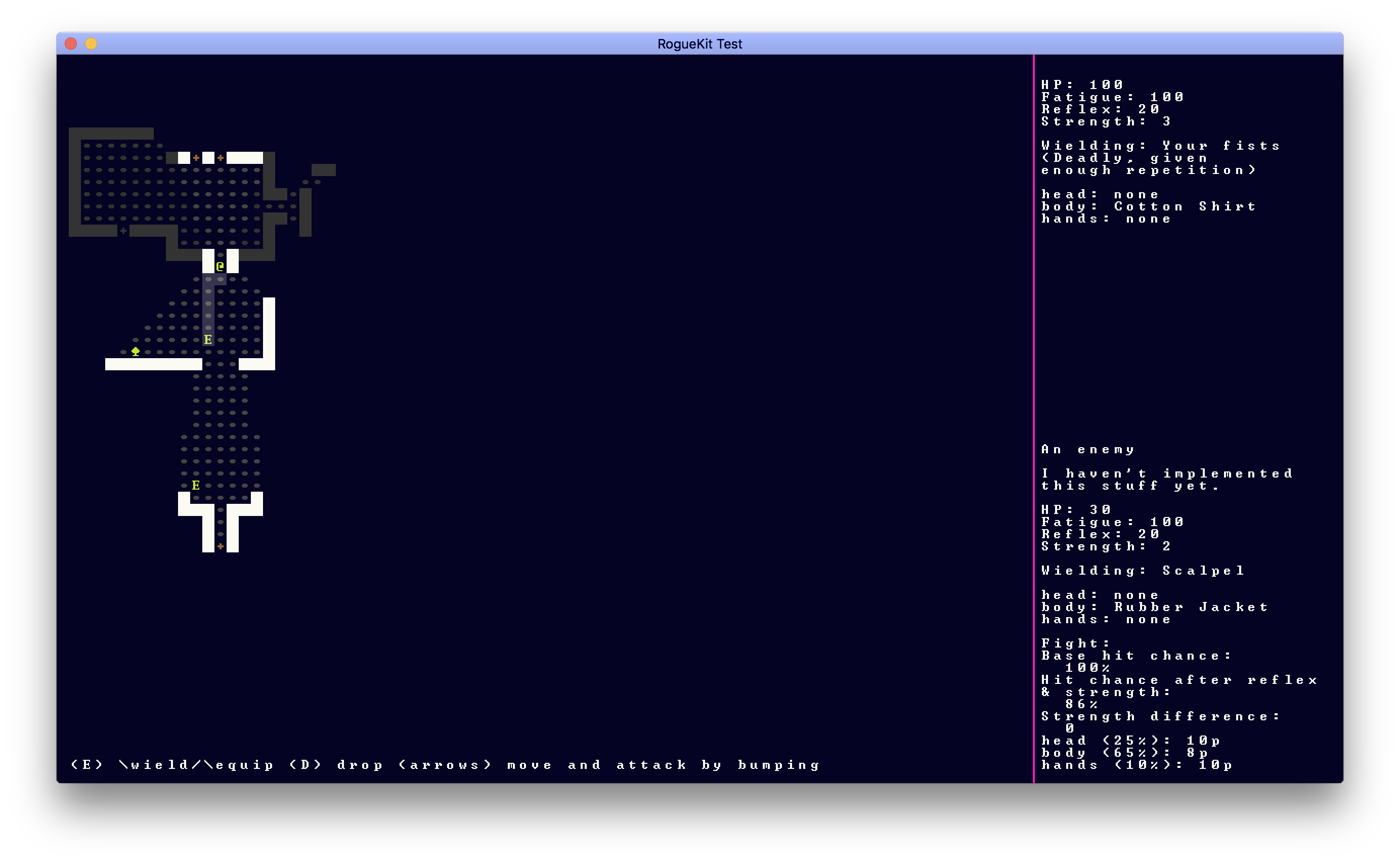
So the player has an 86% chance to hit, and if they hit, they will either do 10 physical damage by hitting the head (25% chance), 8 physical damage by hitting the body (65% chance), or 10 physical damage by hitting the hands (10% chance).
Despite computing outcomes, you can't actually fight in the game yet. That's the first thing I'm doing today!
Files
Get Dr. Hallervorden
Dr. Hallervorden
A roguelike about escaping from a mad scientist's lab
Status | Prototype |
Author | irskep |
Genre | Role Playing |
Tags | Seven Day Roguelike Challenge, ascii, Roguelike |
Languages | English |
Accessibility | Color-blind friendly, High-contrast |
More posts
- 7DRL: DoneMar 11, 2018
- 7DRL Day 6: Five maps total, UI improvements, animationsMar 09, 2018
- 7DRL Day 5: My First Themed MapsMar 08, 2018
- 7DRL Day 4: Enemy types, map configurationMar 07, 2018
- 7DRL Day 3: Combat worksMar 06, 2018
- 7DRL day 1: ItemsMar 04, 2018
- Preparation for Dr. HallervordenMar 03, 2018
Leave a comment
Log in with itch.io to leave a comment.